In this article, we will learn about the WHERE statement in SQL. This statement is used to filter the results of a SELECT, UPDATE, or DELETE statement based on specified conditions in SQL.
The syntax of the WHERE clause is as follows
[ WHERE <search_condition> ]
Statement 1. Finding a row by using a simple equality
How to find a specific row in a database table using a simple equality comparison. To do this, you will need to know the name of the table and the name of the column that you want to compare against. For example, if you have a table called "OrderDetails" with columns for "OrderName," "orderAddress," and "OrderDate," you could find a specific customer by searching for their OrderName like this.
SELECT OrderName, orderAddress,OrderDate
FROM OrderDetails
WHERE OrderName = 'Apple' ;
The SQL query you provided selects the columns 'OrderName,' 'orderAddress,' and 'OrderDate' from the 'OrderDetails' table where the 'OrderName' is equal to 'Apple.' Assuming that the 'OrderName' column contains the name of the product being ordered and the 'orderAddress' column contains the address where the order is being delivered, this query would return the 'OrderName,' 'orderAddress,' and 'OrderDate' information for all orders of the product 'Apple.'
Note. that the syntax and exact query result would depend on the specific schema and data in the 'OrderDetails' table.
Output
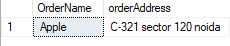
Statement 2. Finding rows by using a comparison operator
Using simple equality, you can use the following SQL query to find a row in a database table.
SELECT OrderName, OrderAddress
FROM OrderDetails
WHERE OrderId <= 5;
The SQL query you provided selects the OrderName and OrderAddress columns from the OrderDetails table where the OrderId is less than or equal to 5. The WHERE clause filters the rows of the table based on a specified condition. In this case, the condition is OrderId <= 5, which means that only rows where the OrderId column has a value less than or equal to 5 will be returned.
The result of this query will be a table containing the OrderName and OrderAddress values for the rows where the OrderId is less than or equal to 5. The number of rows returned will depend on how many rows in the OrderDetails table satisfy the condition.
Output
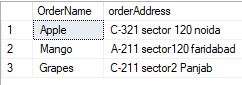
Statement 4. Finding rows that must meet several conditions
To find rows that must meet several conditions in SQL, you can use the AND operator in the WHERE clause. The AND operator allows you to combine two or more conditions that must be true for a row to be included in the query result.
SELECT OrderName, orderAddress
FROM OrderDetails
WHERE OrderId <= 1 AND OrderName LIKE '%App%' AND orderAddress LIKE '%N%';
This SQL query selects the OrderName and orderAddress columns from the OrderDetails table, where the OrderId is less than or equal to 1, the OrderName contains the substring "App," and the orderAddress contains the letter "N". In other words, it will return the order details for any orders with an OrderId of 1 or less, a name containing "App," and an address containing the letter "N". Please note that the syntax and functionality of this query may vary depending on the specific SQL implementation being used.
Output
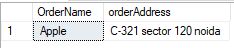
Statement 5. Finding rows that are in a list of values
You can use a SQL query with the IN operator to find rows in a list of values. Here's an example:
Suppose you have a table called OrderDetails with columns OrderName and Address, and you want to find all rows where the name is in a list of values ('Apple,' 'Mango,' 'Banna'). You can use the following query.
SELECT OrderName, orderAddress
FROM OrderDetails
WHERE OrderName IN ('Apple', 'Mango', 'Banna');
SQL query that selects the "OrderName" and "orderAddress" columns from the "OrderDetails" table, but only for orders with an "OrderName" of 'Apple,' 'Mango,' or 'Banna.' Here is the revised code with the corrected spelling for "Banana."
Output
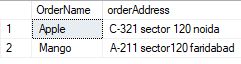
This query will return a result set containing the "OrderName" and "orderAddress" columns for all orders with an "OrderName" of 'Apple,' 'Mango,' or 'Banana.'
Conclusion
This article taught us learned the basics of the SQL Where Statement.
FAQs
Q- What is the WHERE statement in SQL?
A- The WHERE statement is a clause in SQL that is used to filter data based on a specific condition. It is used in conjunction with the SELECT statement to retrieve data from one or more tables in a database.
Q- What are some common operators used in the WHERE statement?
A- Some common operators used in the WHERE statement include
Equals (=)
Not equals (<> or !=)
Less than (<)
Greater than (>)
Less than or equal to (<=)
Greater than or equal to (>=)
LIKE (used for pattern matching)
IN (used to check if a value is in a list)
Q- Can you use multiple conditions in a WHERE statement?
A- Yes, you can use multiple conditions in a WHERE statement by using logical operators such as AND, OR, and NOT.
Q- What is the order of precedence for logical operators in a WHERE statement?
A- The order of precedence for logical operators in a WHERE statement is as follows
NOT
AND
OR
HostForLIFEASP.NET SQL Server 2019 Hosting
