
November 2, 2016 08:32 by
Peter
Angular js URL:
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
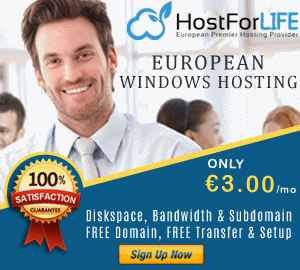
Html tag:
<div ng-app="myApp" ng-controller="myCntrl">
<table>
<thead>
<tr>
<th>
Emp Code.
</th>
<th>
Employee Name
</th>
<th>
Pan No
</th>
</tr>
</thead>
<tr ng-repeat="student in EmployeeList">
<td ng-bind="student.StudentID">
</td>
<td ng-bind="student.StudentName">
</td>
<td ng-bind="student.PanNO">
</td>
</tr>
</table>
</div>
Angular js Script :
<script>
var app = angular.module("myApp", []);
app.controller("myCntrl", function ($scope, $http) {
$scope.fillList = function () {
$scope.EmployeeName = "";
var httpreq = {
method: 'POST',
url: 'Default2.aspx/GetList',
headers: {
'Content-Type': 'application/json; charset=utf-8',
'dataType': 'json'
},
data: {}
}
$http(httpreq).success(function (response) {
$scope.EmployeeList = response.d;
})
};
$scope.fillList();
});
</script>
Asp.net Cs page code:
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.Data;
public partial class Default2 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[System.Web.Services.WebMethod()]
public static List<Employee> GetList()
{
List<Employee> names = new List<Employee>();
DataSet ds = new DataSet();
using (SqlConnection con = new SqlConnection(@"Data Source=140.175.165.10;Initial Catalog=Payroll_290716;user id=sa;password=Goal@12345;"))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.Connection = con;
cmd.CommandText = "select EmpId,Empcode, name,PanNo from EMPLOYEEMASTER order by Name;";
using (SqlDataAdapter da = new SqlDataAdapter(cmd))
{
da.Fill(ds);
}
}
}
if (ds != null && ds.Tables.Count > 0)
{
foreach (DataRow dr in ds.Tables[0].Rows)
names.Add(new Employee(int.Parse(dr["EmpId"].ToString()), dr["name"].ToString(), dr["PanNo"].ToString()));
}
return names;
}
}
public class Employee
{
public int StudentID;
public string StudentName;
public string PanNO;
public Employee(int _StudentID, string _StudentName, string _PanNO)
{
StudentID = _StudentID;
StudentName = _StudentName;
PanNO = _PanNO;
}
}
Whole HTML page:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<form id="form1" runat="server">
<div ng-app="myApp" ng-controller="myCntrl">
<table>
<thead>
<tr>
<th>
Emp Code.
</th>
<th>
Employee Name
</th>
<th>
Pan No
</th>
</tr>
</thead>
<tr ng-repeat="student in EmployeeList">
<td ng-bind="student.StudentID">
</td>
<td ng-bind="student.StudentName">
</td>
<td ng-bind="student.PanNO">
</td>
</tr>
</table>
</div>
<script>
var app = angular.module("myApp", []);
app.controller("myCntrl", function ($scope, $http) {
$scope.fillList = function () {
$scope.EmployeeName = "";
var httpreq = {
method: 'POST',
url: 'Default2.aspx/GetList',
headers: {
'Content-Type': 'application/json; charset=utf-8',
'dataType': 'json'
},
data: {}
}
$http(httpreq).success(function (response) {
$scope.EmployeeList = response.d;
})
};
$scope.fillList();
});
</script>
</form>
</body>
</html>
HostForLIFE.eu AngularJS Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
